How to Add Google Ads gtag.js Tracking to Your Next js Website
June 04, 2024Introduction to gtag.js and Next.js
gtag.js is a JavaScript tagging framework and API developed by Google that simplifies the process of integrating multiple Google products, including Google Ads, into your website. It allows you to send event data to Google Analytics, Google Ads, and other Google services, enabling accurate tracking and analysis of your marketing campaigns.
Benefits of Adding gtag.js to Your Next.js Website
Next.js, on the other hand, is a popular React framework that enables server-side rendering and static site generation. By combining the power of gtag.js with Next.js, you can ensure that your Google Ads data is accurately tracked across your application, helping you make informed marketing decisions and optimize your campaigns for better performance.
Prerequisites for Integrating gtag.js
- A Google Ads account
- Access to your Next.js project
- Basic knowledge of React and Next.js
Before you begin, ensure you have the following:
Step-by-Step Guide Adding Google gtag.js code in Next.js website
Create a Google Ads Account
If you don't already have a Google Ads account, create one in this link.
Obtain Your Google Ads Tracking ID
- Log in to your Google Ads account.
- Click the "Tools & Settings" icon in the upper right corner.
- Under "Measurement", select "Conversions".
- Click on the "+" button to create a new conversion action.
- Follow the prompts to set up your conversion action and obtain your tracking ID (usually starts with `AW-`).
Add Google Ads gtag code to Your Next.js Website using Google Tag Manager
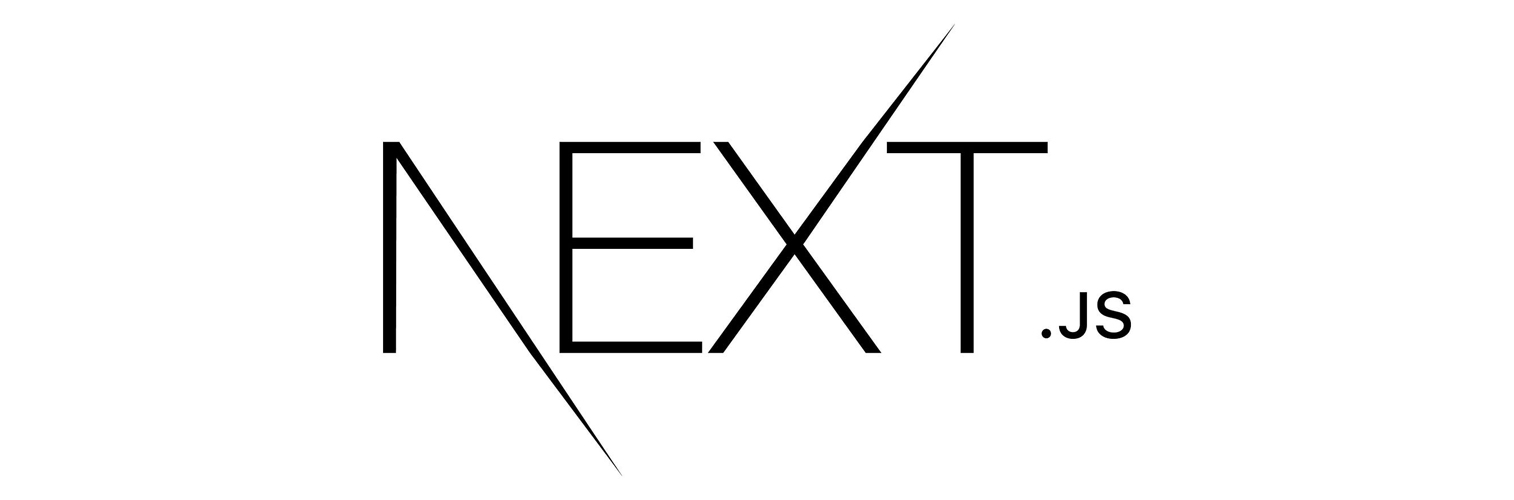
You can add the Google tag for Google Ads to your Next.js project without installing any third-party packages.
Step 1:
Open your Next.js application files, and locate the layout.js file, which is usually located at root/src/app/layout.js if you are using the src and app folders, or root/app/layout.js .
You can find your project structure in this documentation.
Step 2:
Your layout.js might look like this:
import Navbar from './navbar';
import Footer from './footer';
export default function Layout({ children }) {
return (
<>
<Navbar />
<main>{children}</main>
<Footer />
</>
);
}
Step 3:
Since adding the script tag to your head tag can be challenging with all the packages around the community, and _app.js or _document.js might not work in some scenarios, you can directly embed the script tag in layout.js , which will reflect on all pages of the website.
// layout.js
import Script from 'next/script';
import Navbar from './navbar';
import Footer from './footer';
export default function Layout({ children }) {
return (
<html lang="en">
<head>
<Script id="gtm" strategy="afterInteractive">
{`(function(w,d,s,l,i){w[l]=w[l]||[];w[l].push({'gtm.start':
new Date().getTime(),event:'gtm.js'});var f=d.getElementsByTagName(s)[0],
j=d.createElement(s),dl=l!='dataLayer'?'&l='+l:'';j.async=true;j.src=
'https://www.googletagmanager.com/gtm.js?id='+i+dl;f.parentNode.insertBefore(j,f);
})(window,document,'script','dataLayer','GTM-xxxxxxxx');`}
</Script>
</head>
<body>
<Navbar />
<main>{children}</main>
<Footer />
</body>
</html>
);
}
In the above example code,we defined the <html>
and <head>
tags in layout.js
and included the script tag using <Script>
from "next/script" .
Don't forget to import the Script component from "next/script" .
We often get two script tags to add to our website. The first one should be added in the head tag, and the next one should be added immediately after the opening of your body tag. To do this, we can use the same layout.js file. Instead of adding the script directly, we can create a component for this and import it inside the body tag.
Step 4:
Create a component called GoogleTagManager.jsx/tsx in src/app/components/analytics/GoogleTagManager.jsx :
const GoogleTagManager = () => {
return (
<noscript>
<iframe
src="https://www.googletagmanager.com/ns.html?id=GTM-xxxxxxxx" // Place your GTM code here
height="0"
width="0"
style={{ display: 'none', visibility: 'hidden' }}
></iframe>
</noscript>
);
};
export default GoogleTagManager;
Step 5:
Now import this component in your layout.js like below:
import Script from 'next/script';
import Navbar from './navbar';
import Footer from './footer';
import GoogleTagManager from '@/components/analytics/GoogleTagManager';
export default function Layout({ children }) {
return (
<html lang="en">
<head>
<Script id="gtm" strategy="afterInteractive">
{`(function(w,d,s,l,i){w[l]=w[l]||[];w[l].push({'gtm.start':
new Date().getTime(),event:'gtm.js'});var f=d.getElementsByTagName(s)[0],
j=d.createElement(s),dl=l!='dataLayer'?'&l='+l:'';j.async=true;j.src=
'https://www.googletagmanager.com/gtm.js?id='+i+dl;f.parentNode.insertBefore(j,f);
})(window,document,'script','dataLayer','GTM-xxxxxxxx');`} // Place your GTM code here
</Script>
</head>
<body>
<GoogleTagManager />
<Navbar />
<main>{children}</main>
<Footer />
</body>
</html>
);
}
Import the component immediately after the opening of your body tag.
Build and run your application. The script tag will reflect on your website.
Step by Step Guide: Add Google Ads gtag.js code in Next.js website using Google Tag Manager package
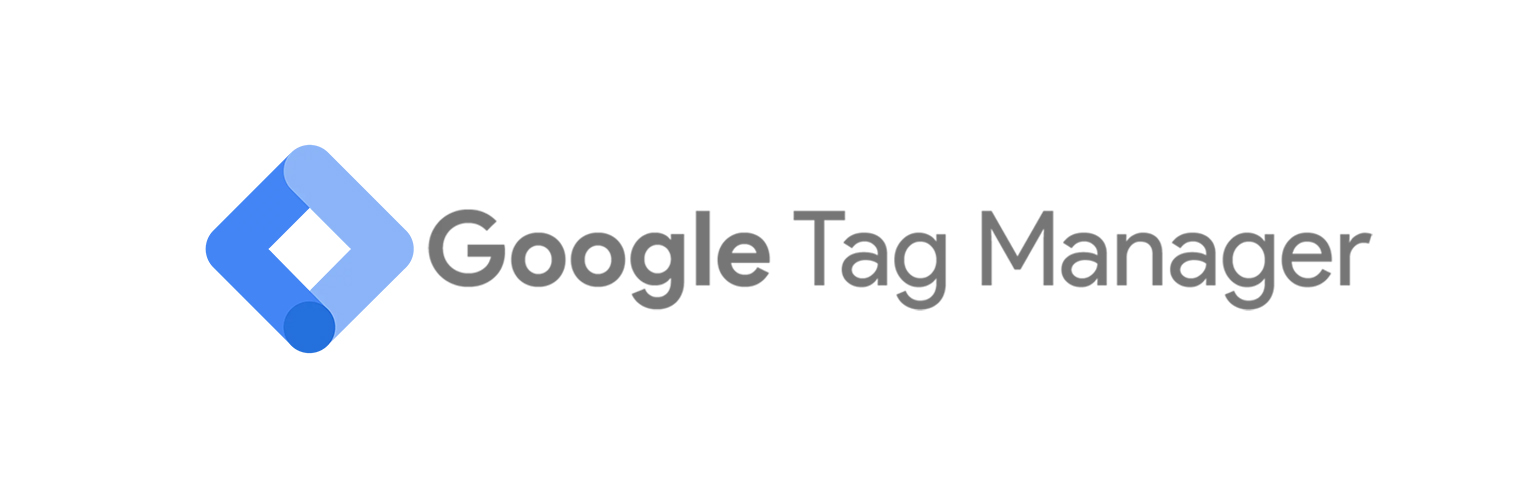
Step 1:
Install gtag.js: Open your terminal and navigate to your Next.js project. Install the necessary package.
npm install --save react-gtm-module
Step 2:
Create a Utility for gtag: Create a new file in your `lib` directory named `gtag.js` .
// lib/gtag.js
export const GA_TRACKING_ID = 'YOUR_TRACKING_ID'; // Replace with your Google Ads tracking ID
// Function to send page views to Google Ads
export const pageview = (url) => {
window.gtag('config', GA_TRACKING_ID, {
page_path: url,
});
};
// Function to send custom events to Google Ads
export const event = ({ action, category, label, value }) => {
window.gtag('event', action, {
event_category: category,
event_label: label,
value: value,
});
};
Step 3:
Update the _app.js File: Modify your `_app.js` file to include gtag.js and set up page view tracking
// pages/_app.js
import { useEffect } from 'react';
import { useRouter } from 'next/router';
import * as gtag from '../lib/gtag';
function MyApp({ Component, pageProps }) {
const router = useRouter();
useEffect(() => {
const handleRouteChange = (url) => {
gtag.pageview(url);
};
router.events.on('routeChangeComplete', handleRouteChange);
return () => {
router.events.off('routeChangeComplete', handleRouteChange);
};
}, [router.events]);
return <Component {...pageProps} />;
}
export default MyApp;
Step 4:
Add gtag.js Script to _document.js: Add the gtag.js script to your Next.js application by modifying the `_document.js` file.
// pages/_document.js
import Document, { Html, Head, Main, NextScript } from 'next/document';
import { GA_TRACKING_ID } from '../lib/gtag';
class MyDocument extends Document {
render() {
return (
<Html>
<Head>
{/* Global Site Tag (gtag.js) - Google Ads */}
<script async src={`https://www.googletagmanager.com/gtag/js?id=${GA_TRACKING_ID}`} />
<script
dangerouslySetInnerHTML={{
__html: ``
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', '${GA_TRACKING_ID}', {
page_path: window.location.pathname,
});
`,}}}
/>
</Head>
<body>
<Main />
<NextScript />
</body>
</Html>
);
}
}
export default MyDocument;
Verifying Your Setup
After implementing gtag.js in your Next.js application, you should verify that everything is working correctly.
- Google Tag Assistant: Use the Google Tag Assistant Chrome extension to ensure your tags are firing correctly.
- Google Ads: Check the "Conversions" section in your Google Ads account to see if data is being recorded.
Troubleshooting Common Issues
- - Tags Not Firing: Ensure that your tracking ID is correctly set and that the gtag.js script is properly included in your `_document.js` file.
- - No Data in Google Ads: Check for any JavaScript errors in your browser’s console that might indicate issues with your implementation.
Conclusion
Adding gtag.js for Google Ads in your Next.js application is a straightforward process that can significantly enhance your ad tracking and optimization capabilities. By following this guide, you can ensure that your Google Ads data is accurately tracked, helping you make better marketing decisions and improve your return on investment.
Go Back